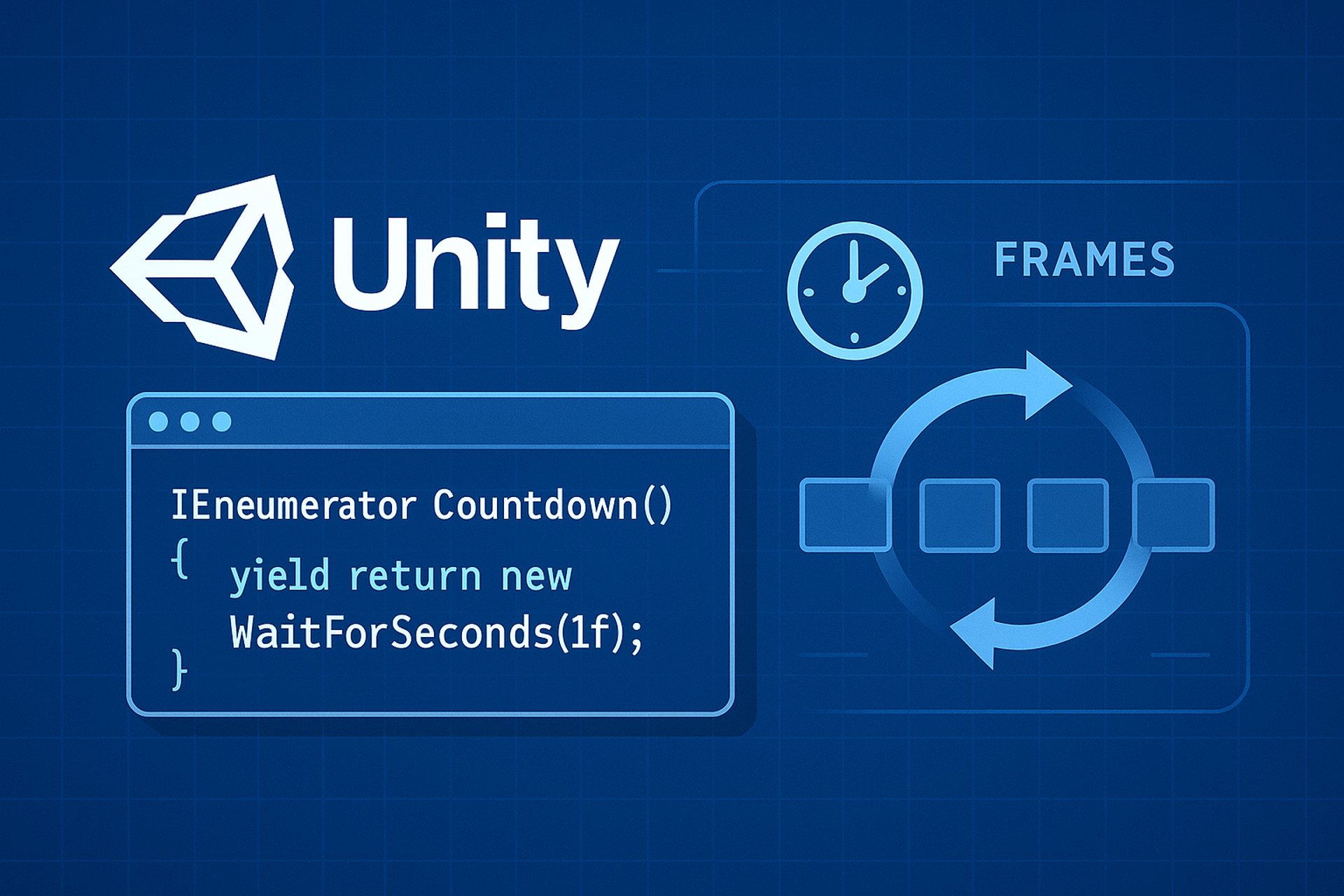
Using Coroutines in Unity: A Practical Guide with Crazy 8 Ball Pot
Discover how Unity Coroutines can simplify time-based logic and asynchronous tasks in your game. In this beginner-friendly guide, you’ll learn what coroutines are, why and when to use them, see a simple countdown example in action, weigh their pros and cons, and explore alternative approaches for smooth, non-blocking workflows.
GAME DEVELOPMENT
CLEF Games
A coroutine in Unity is a special function that allows you to pause execution and resume it later, without blocking the main game loop.
Technically, coroutines are methods that return an IEnumerator and use yield statements to wait for events like time delays, frames, or custom conditions. They run alongside Unity’s Update loop, making them ideal for sequencing actions over time without complicated state machines.
Why Are Coroutines Used?
In real-time games, you often need to wait or spread work across multiple frames—think animations, timed events, or smooth transitions—without freezing the entire game. Coroutines let you express these sequences in clear, linear code instead of splitting logic into flags and timers in Update().
For example, in Crazy 8 Ball Pot, coroutines handle the ball-launch delay, camera shakes after a hit, and UI pop-up timings, creating a smooth user experience without blocking input or other game systems.
Create a Coroutine Method
Define a method returning IEnumerator, using yield return to pause:IEnumerator LaunchBallRoutine(float delay) {
// Wait before launching the next ball
yield return new WaitForSeconds(delay);
LaunchNextBall();
}
Start the Coroutine
Call it from another script or event:StartCoroutine(LaunchBallRoutine(1.5f));
Chaining Actions
You can sequence multiple waits and actions:IEnumerator HitFeedback() {
// Flash the ball red
ballRenderer.material.color = Color.red;
yield return new WaitForSeconds(0.2f);
// Revert color
ballRenderer.material.color = Color.white;
// Shake camera
yield return ShakeCamera(0.5f);
}
Stopping a Coroutine
If you need to cancel it before completion:StopCoroutine(nameof(LaunchBallRoutine));
Advantages and Disadvantages
Advantages
Readability: Write time-based sequences in straightforward, linear code.
Non-Blocking: Coroutines won’t freeze the game; other systems continue running.
Flexibility: Easily wait for seconds, frames, or custom conditions (e.g., yield return new WaitUntil(() => playerHealth <= 0)).
Disadvantages
Debugging Complexity: Stalled or endlessly running coroutines can be tricky to trace.
Lifecycle Dependency: If the GameObject or MonoBehaviour is destroyed, running coroutines stop without warning.
Performance Overhead: Starting thousands of coroutines simultaneously can incur overhead; use sparingly for small, timed tasks.
Alternatives
Update Method with Timers: Manually track elapsed time in Update(). Good for simple cases but can clutter your loop logic.
Invoke and InvokeRepeating: Unity’s built-in delayed calls (Invoke("MethodName", 1.5f)). Less flexible for complex sequences.
Async/Await with UniTask or Task: Modern C# async patterns (with third-party libraries) for truly asynchronous operations. Cleaner error handling but requires extra setup.
Tweening Libraries (DOTween): For animations and timed value changes, Tween engines offer rich features and chaining with minimal code.
Coroutines are a powerful Unity feature when used appropriately. As you build your next game, leverage coroutines for any sequence that unfolds over time but remember to manage and monitor them carefully to avoid hidden bugs or performance issues.